Table of Contents
- Getting Started
- EO.Pdf
- Overview
- Installation and Deployment
- Using HTML to PDF
- Using PDF Creator
- Using PDF Creator
- Getting Started
- Advanced Formatting Techniques
- Interactive Features
- Low Level Content Objects
- Working with Existing PDF Files
- Using in Web Application
- Advanced Topics
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Common Topics
- Reference
Using Table |
Overview
You can create a two dimensional table with AcmTable object.
Creating a Table
The following code demonstrates how to create a simple table with 2 rows and 2 columns.
//Create a table object with two columns. The //first column is 2 inch width and the second //column is 3 inch width AcmTable table = new AcmTable(2f, 3f); //Create the first row and cells AcmTableRow row = new AcmTableRow(); AcmTableCell cell1 = new AcmTableCell(new AcmText("Cell 1")); AcmTableCell cell2 = new AcmTableCell(new AcmText("Cell 2")); //Add the first row and cells table.Rows.Add(row); row.Cells.Add(cell1); row.Cells.Add(cell2); //Create the second row and cells row = new AcmTableRow(); cell1 = new AcmTableCell(new AcmText("Cell 3")); cell2 = new AcmTableCell(new AcmText("Cell 4")); //Add the second row and cells table.Rows.Add(row); row.Cells.Add(cell1); row.Cells.Add(cell2);
Cell Padding and Spacing
You can set an AcmTable's CellPadding and CellSpacing to reserve space inside the cell or in between cells. If CellPadding is set, then it overrides any padding values that may present on the cell object. If CellSpacing is set, then it overrides all cell's margin value with half of the set value.
Alternatively, you can also set Margin or Padding on each cell directly to reserve space inside or in between cells.
Table Grid Lines
A table can optionally have horizontal grid line, vertical grid line or both. Table grid lines are drawn in between table cells and may coexist with table cell borders. The following code demonstrates how to enable table grid lines:
//Set the grid line type table.GridLineType = AcmGridLineType.All; //Set the grid line style, color and width table.GridLineInfo = new AcmLineInfo( AcmLineStyle.Solid, System.Drawing.Color.Black, 0.01f);
Optionally you can also set borders on each AcmTableCell object to create lines around a cell or horizontal/vertical line along a row of cells or columns. Note this only works on AcmTableCell. Margin, border, and padding settings on AcmTableRow object are always ignored.
Column Span and Cell Span
A cell can span into multiple columns or rows. The following diagram illustrates the result layout when the first cell spans into two rows and two columns.
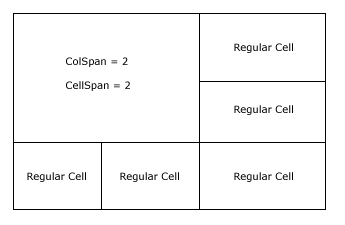
To span a cell into multiple columns or rows, simply set the cell's ColSpan or RowSpan to a value greater than 1. Note that once you have cells spanning into multiple cells, the actual number of AcmTableCell objects in a row would be less than the number of columns in the table. RowSpan has the same effect. The code to create a table with the above layout would be:
//Create a table with 3 columns, each column is 2 inch AcmTable table = new AcmTable(2f, 2f, 2f); table.CellPadding = 0.05f; //Create the first row AcmTableRow row = new AcmTableRow(); //Create the first cell which spans into 2 columns and 2 rows AcmTableCell cell = new AcmTableCell( new AcmText("Spanning Cell")); cell.ColSpan = 2; cell.RowSpan = 2; row.Cells.Add(cell); //Create the "third" cell. Note we do not need a "second" //cell because the first cell spans two cells cell = new AcmTableCell(new AcmText("Regular Cell")); row.Cells.Add(cell); //Add the row into the table. Note this row only has //two AcmTableCell objects table.Rows.Add(row); //Create the second row row = new AcmTableRow(); //Create the "third" cell for the second row. Note //that we do not need to a "first" or "second" cell //for this row because the cell above takes two cells //and spans into this row cell = new AcmTableCell(new AcmText("Regular Cell")); row.Cells.Add(cell); //Add the row into the table. Note this row only //has a single AcmTableCell object table.Rows.Add(row); //Create the third row row = new AcmTableRow(); //Create the first cell cell = new AcmTableCell(new AcmText("Regular Cell")); row.Cells.Add(cell); //Create the second cell cell = new AcmTableCell(new AcmText("Regular Cell")); row.Cells.Add(cell); //Create the third cell cell = new AcmTableCell(new AcmText("Regular Cell")); row.Cells.Add(cell); //Add the row into the table. This row is normal and has //all three AcmTableCell objects. table.Rows.Add(row); //Set the grid line type table.GridLineType = AcmGridLineType.All; //Set the grid line style, color and width table.GridLineInfo = new AcmLineInfo( AcmLineStyle.Solid, System.Drawing.Color.Black, 0.01f);
Auto Fit Column
An auto-fit column is a column that automatically takes the maximum available width. For example, if the page is 6 inch width and the table have two fixed width column both of 1 inch wide, then an auto-fit column would be 4 inch width if no margin, border or padding are set.
To specify an auto-fit column, pass -1 as its column width when creating the table. For example:
//Create a table with three columns. The first and the //third column are both 1 inch width. The second column //would automatically adjust so that the whole table takes //the full available width AcmTable table = new AcmTable(1f, -1f, 1f);